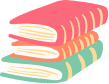
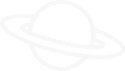
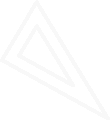
Blog Details
- Home
- Blog page
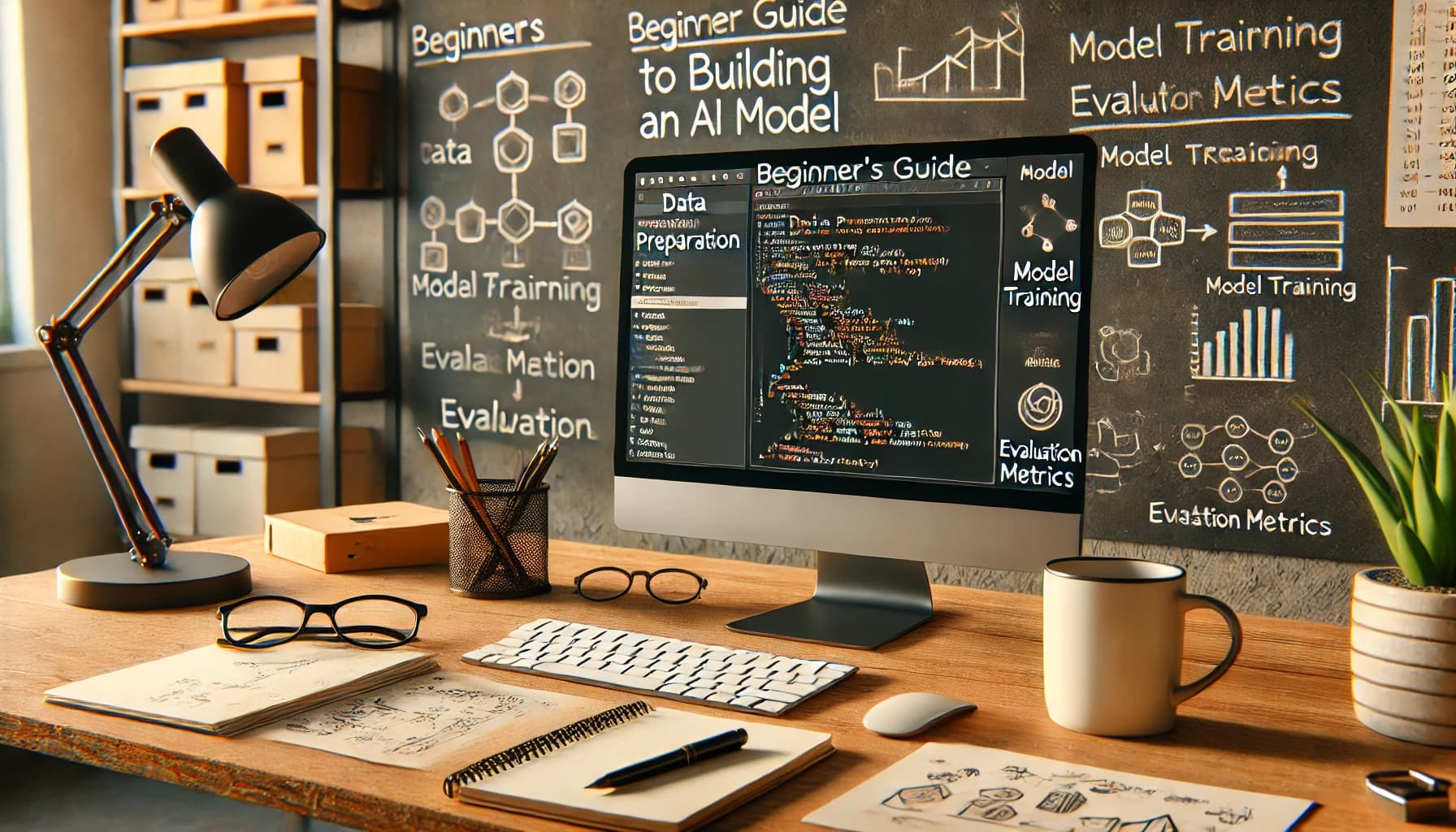
Building Your First AI Model: Step-by-Step for Beginners
Artificial Intelligence (AI) is changing the world at a fast pace, impacting everything from healthcare to entertainment. If you’re interested in building your own AI model, you’re not alone! Getting started may seem overwhelming, but with some guidance, you’ll find it’s entirely doable. This beginner’s guide will take you through the essential steps to build your first machine learning model.
We’ll start with a brief overview of machine learning, dive into essential terminology, and walk through creating a simple model. By the end of this post, you’ll have a functional AI model and a clear understanding of the steps involved.
Step 1: Understanding the Basics of Machine Learning
Before building your model, let’s clarify some core concepts:
➡️Machine Learning (ML): A subset of AI, where algorithms learn from data to make predictions or decisions without explicit programming.
➡️Supervised Learning: The model learns from labeled data (data with correct answers).
➡️Unsupervised Learning: The model learns patterns without labeled data.
➡️Dataset: A collection of data points used for training and testing the model.
➡️Features and Labels: Features are input variables, while labels are the target or output variable we want the model to predict.
For your first model, we’ll use supervised learning and create a simple model that predicts a target value based on input features.
Step 2: Choosing a Problem to Solve
Choose a problem that’s simple, measurable, and has readily available data. A common example for beginners is predicting housing prices based on features like square footage, number of bedrooms, and location. In this example, our goal is to predict house prices based on historical data.
Step 3: Preparing the Data
Data is the foundation of any AI model, so let’s start by gathering and preparing it. You can find free datasets on platforms like Kaggle or UCI Machine Learning Repository.
For a house price prediction model, you’ll need a dataset containing information about various houses, including features (square footage, number of bedrooms, etc.) and labels (the sale price).
Key Steps in Data Preparation:
1. Collect the Data: Download the dataset and load it into your environment. For example, in Python, you can use Pandas to load CSV data.
import pandas as pd
data = pd.read_csv("house_prices.csv")
2. Inspect the Data: Check for any missing values or inconsistencies, which may need to be fixed for accurate model training.
print (data.head())
print (data.info())
3. Clean the Data: Handle any missing values by either removing them or filling them with the median or mean. Ensure all features are in numerical form, as ML algorithms typically work with numerical data.
4. Feature Selection: Select the features you think are most predictive of the label (e.g., square footage and number of bedrooms for predicting house prices). This can be done through trial and error or statistical methods.
Step 4: Splitting the Data
To evaluate the model’s performance, you’ll need to split the data into two sets:
➡️Training Set: Used to train the model.
➡️Test Set: Used to evaluate how well the model can generalize to new data.
A common split ratio is 80% for training and 20% for testing.
from sklearn.model_selection import train_test_split
X = data[['SquareFootage', 'Bedrooms']]
y = data['Price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 5: Choosing and Training a Model
Setting Up the Model
1. Import the Linear Regression model from the scikit-learn library.
2. Initialize the model.
3. Train it on the training data.
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
After running this code, the model will have learned a mathematical relationship between the features and the target price based on the training data.
Step 6: Evaluating the Model
Once trained, it’s time to test the model’s accuracy on the test set. The model will predict prices based on the test features, and we’ll compare these predictions to the actual prices to gauge accuracy.
Common Evaluation Metrics:
1. Mean Absolute Error (MAE): The average absolute difference between predicted and actual values.
2. Mean Squared Error (MSE): The average squared difference between predicted and actual values.
from sklearn.metrics import mean_absolute_error, mean_squared_error
y_pred = model.predict(X_test)
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
print("Mean Absolute Error:", mae)print("Mean Squared Error:", mse)
A lower MAE and MSE indicates a more accurate model.
Step 7: Tuning the Model (Optional)
Hyperparameter tuning can help optimize the model. While linear regression has fewer parameters to tweak, you can explore other algorithms like Decision Trees or Random Forests for more complex models.
For instance, using a Grid Search to test different hyperparameter values can refine your model’s performance.
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestRegressor
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20, 30]
}
grid_search = GridSearchCV(RandomForestRegressor(), param_grid, cv=3)
grid_search.fit(X_train, y_train)
print("Best parameters:", grid_search.best_params_)
Step 8: Deploying the Model
Once you’re satisfied with your model, you can deploy it to make predictions on new data. In production, the model could take new house feature data and predict the price instantly.
For deployment, options include saving the model as a file (using joblib or pickle) or deploying it to a cloud service such as AWS or Google Cloud Platform (GCP).
import joblib
joblib.dump(model,"house_price_model.joblib")
loaded_model = joblib.load("house_price_model.joblib")
Conclusion
Congratulations! You’ve built and trained your first machine learning model. Creating a model from scratch may seem challenging, but by breaking down each step, you can see it’s manageable and incredibly rewarding. You can apply this framework to any other ML project you want to try.
As you continue your AI journey, remember that each model you build is a stepping stone to more advanced projects. Keep experimenting, learning, and improving your skills—you’re on your way to becoming an AI expert!
Search here
Recent Post
Photo Gallery
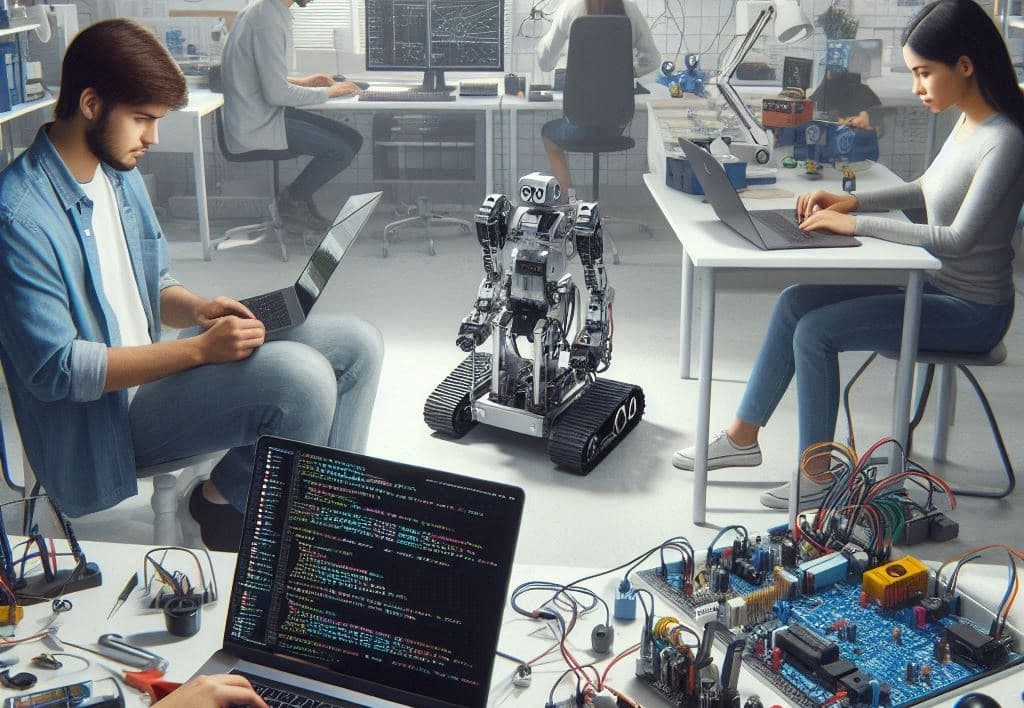
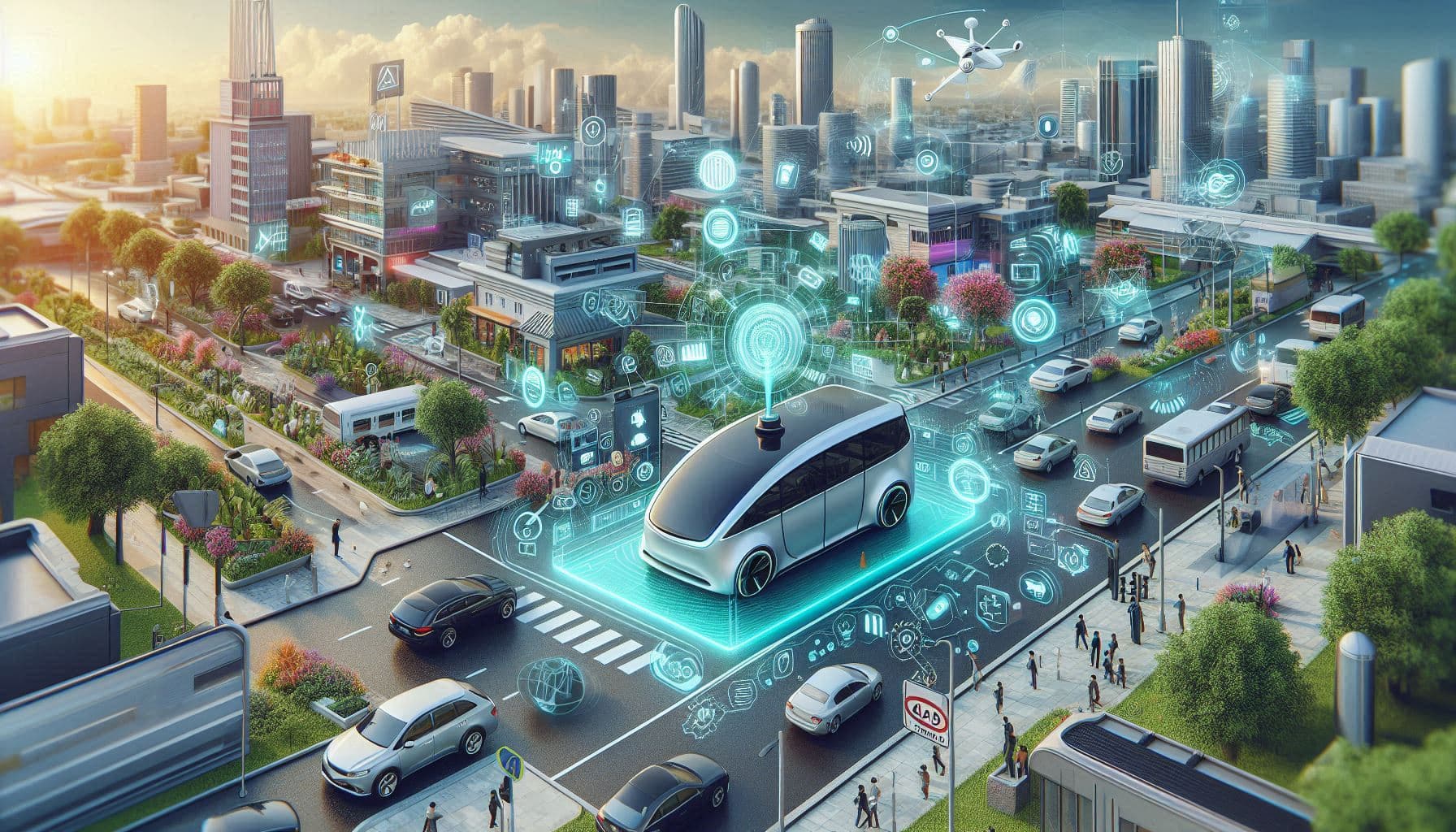
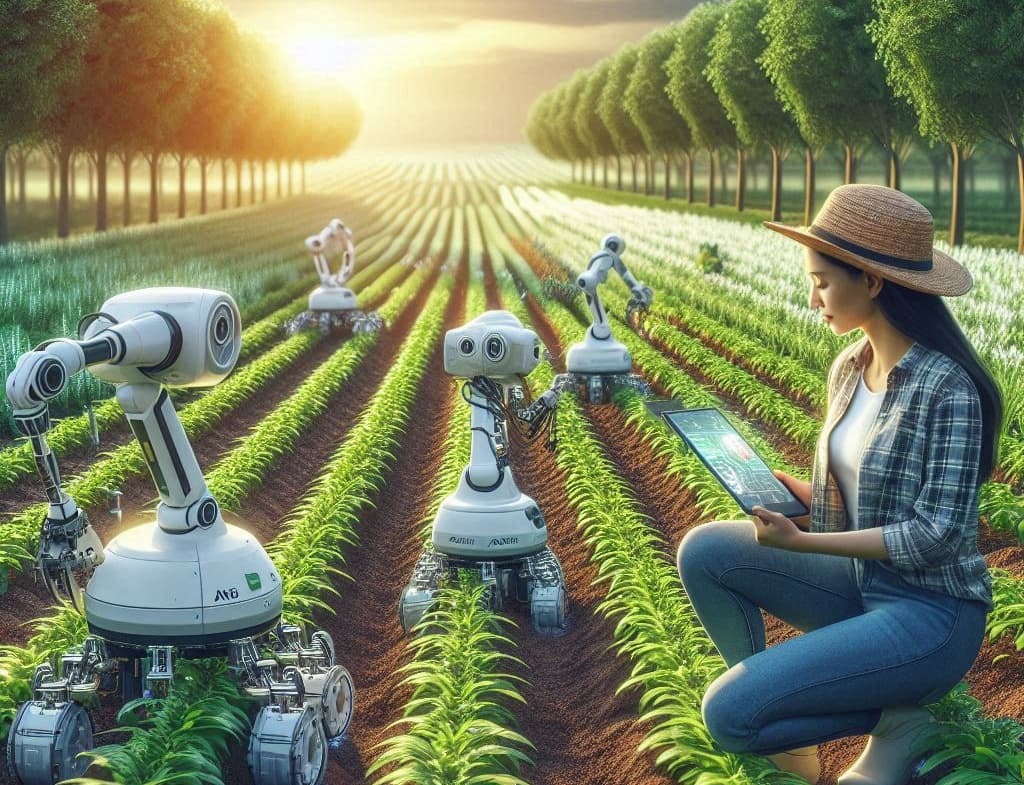
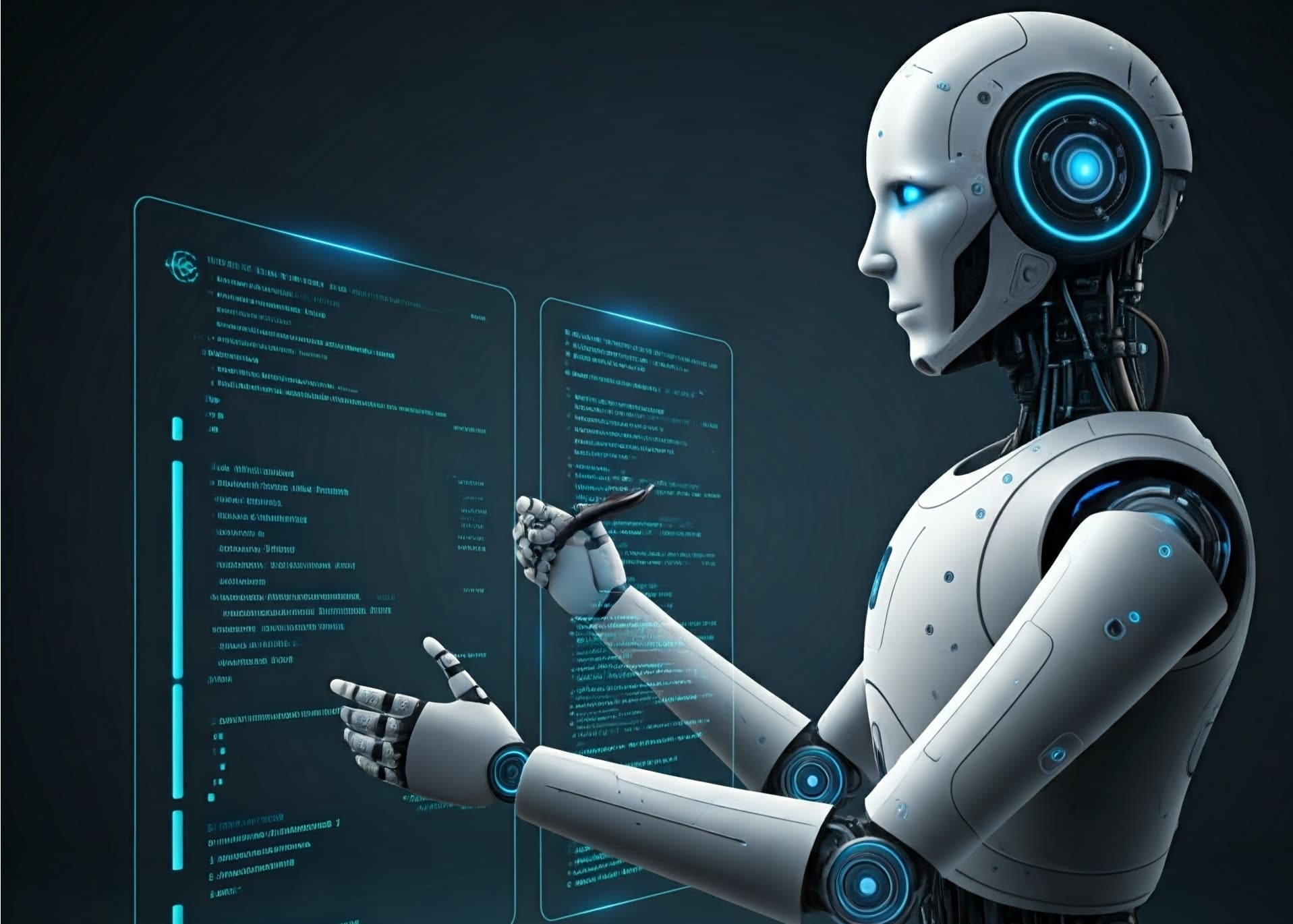
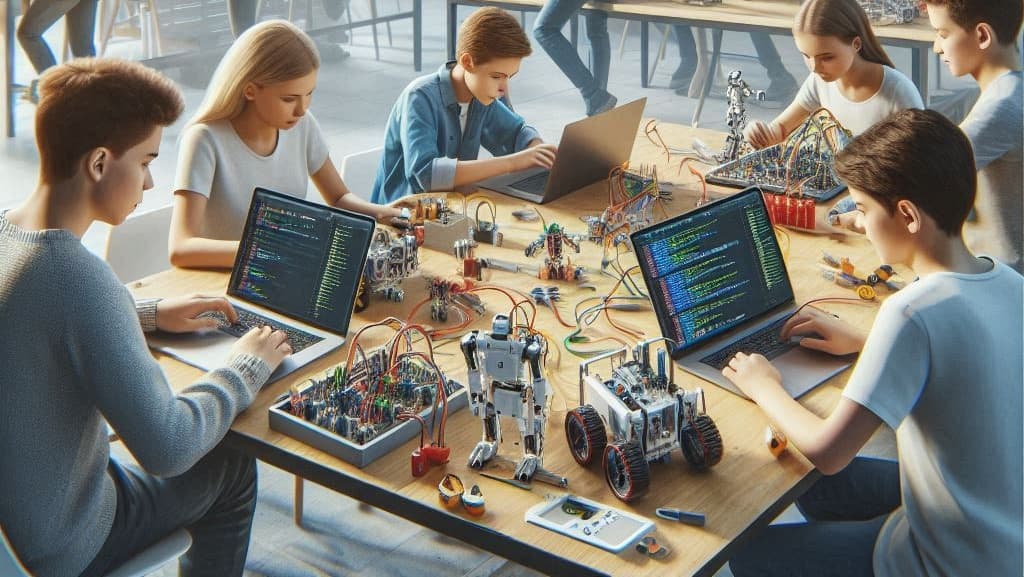
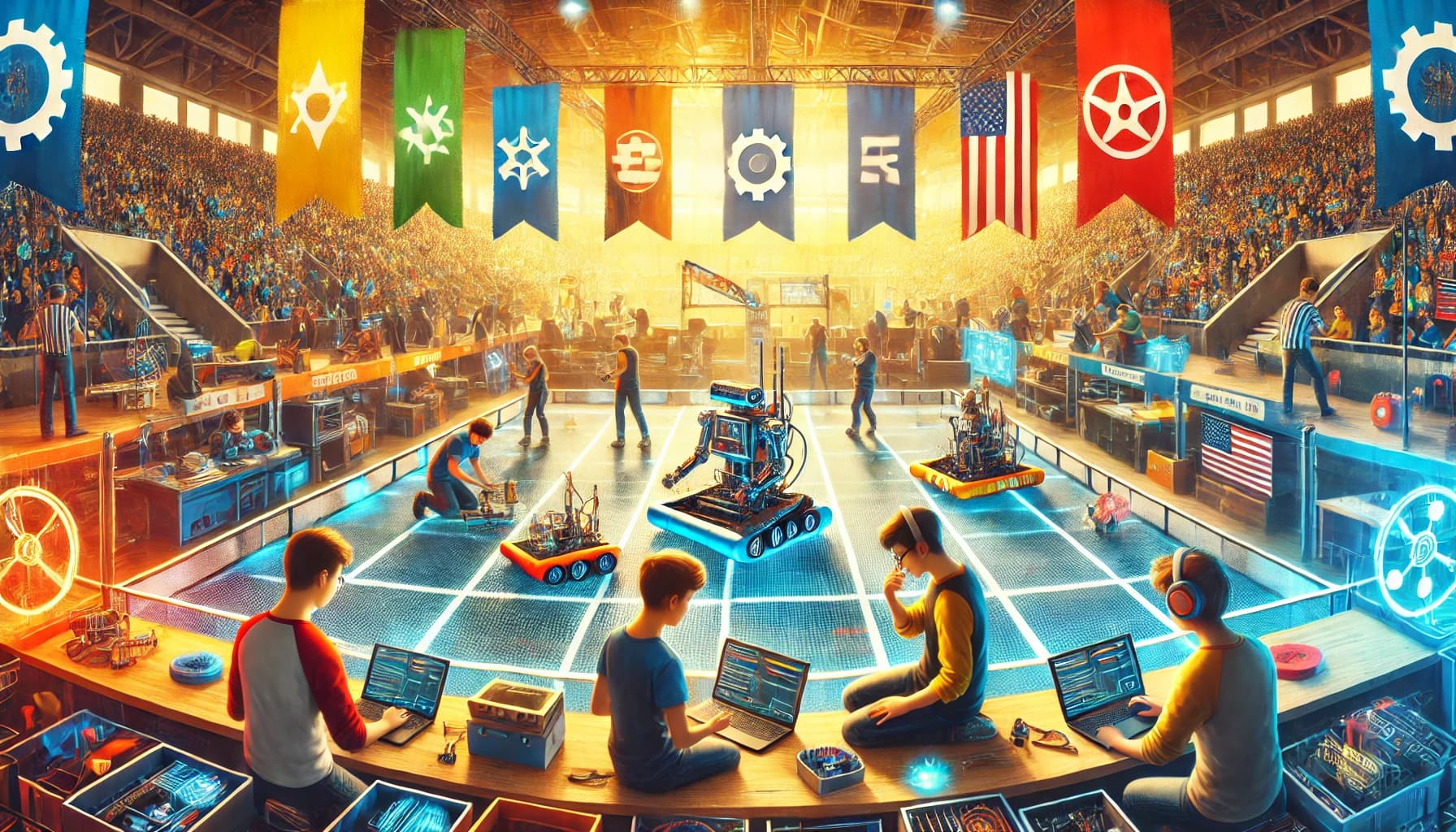